Professional List
The Professional List is a list of all professionals available for the currently authenticated user, allowing access to chats and professional profiles.
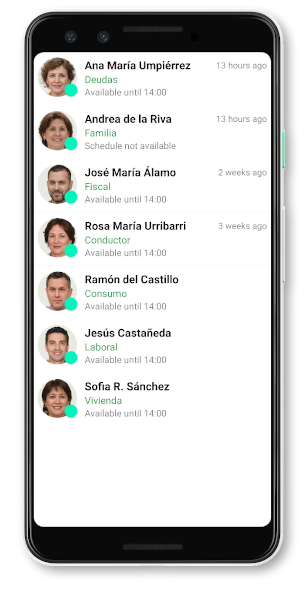
#
IntegrationThere are three ways to integrate the professional list into your project:
#
1. Add ProfessionalList Fragment in Your LayoutTo integrate the professional list directly within your UI, add the following XML to your layout:
Attributes
mlIncludedSpecialities
#
Specifies which specialties should be included in the professional list. If not provided, all specialties will be included. The available specialties are: general, fiscal, debt, driving, work, family, housing, and customer.
mlExcludedSpecialities
#
Specifies which specialties should be excluded in the professional list. If not provided, no specialties will be excluded. The available specialties are: general, fiscal, debt, driving, work, family, housing, and customer.
mlEnableSwipeRefresh
#
Enables or disables the swipe-to-refresh functionality in the professional list. The default value is true.
Public Methods
setTopBanner(@Composable (() -> Unit)?)
#
Sets a top banner for the professional list using a Jetpack Compose composable.
Parameters:
bannerContent
: A composable function representing the banner content.
setTopBanner(view: View?)
#
Sets a top banner using a traditional Android View
.
Parameters:
view
: AView
to be used as the banner.
setDivider(@Composable (() -> Unit)?)
#
Sets a custom divider within the professional list using a Jetpack Compose composable.
Parameters:
dividerContent
: A composable function representing the divider.
setDivider(view: View?)
#
Sets a custom divider using a traditional Android View
.
Parameters:
view
: AView
to be used as the divider.
setDividerIndex(index: Int?)
#
Sets the position where the divider should be placed.
Parameters:
index
: AnInt?
representing the index of the divider placement. Ifnull
, the divider is placed over the first inaccessible professional.
setProfessionalListListener(professionalListListener: ProfessionalListListener?)
#
Sets a listener to handle interactions with the professional list.
Parameters:
professionalListListener
: An instance ofProfessionalListListener
to receive callbacks.
setIncludeSpecialities(specialities: List<Speciality>?)
#
Specifies which specialties should be included in the professional list.
Parameters:
specialities
: A list ofSpeciality
objects to be included.
setExcludeSpecialities(specialities: List<Speciality>?)
#
Specifies which specialties should be excluded from the professional list.
Parameters:
specialities
: A list ofSpeciality
objects to be excluded.
setEnableSwipeRefresh(enable: Boolean)
#
Enables or disables the swipe-to-refresh functionality in the professional list. The default value is true.
Parameters:
enable
: ABoolean
indicating whether swipe refresh should be enabled.
ProfessionalListListener Implementation
We strongly recommend implementing the ProfessionalListListener
for a better integration experience in your app.
The onProfessionalClick()
method lets you control chat openings according to your business logic. The hasAccess
flag indicates whether the authenticated user has access to chats (i.e., they have been activated via the S2S User API).
#
2. Launch Professional List ActivityAlternatively, you can use the following method to launch an activity containing only the professional list view.
openProfessionalList(context: Context, toolbarImageResource: Int?)
#
Launches an activity containing only the professional list view.
Parameters:
context
: The current context.toolbarImage
: A drawable resource ID for the toolbar image.
#
3. Use ProfessionalsScreen in Jetpack ComposeFor Jetpack Compose users, you can integrate the professional list using ProfessionalsScreen
.
#
Compose View Parameters:modifier: Modifier
#
An optional Modifier
to customize the layout.
includeSpecialities: List<Speciality>
#
Specifies which specialties should be included in the professional list.
excludeSpecialities: List<Speciality>
#
Specifies which specialties should be excluded from the professional list.
enablePullToRefresh: Boolean
#
Enables or disables the swipe-to-refresh functionality in the professional list.
onProfessionalClick: (Professional, hasAccess: Boolean) -> Unit
#
A lambda function triggered when a professional is clicked.
onProfessionalAvatarClick: (String) -> Unit
#
A lambda function triggered when a professional avatar is clicked.
onListLoaded: () -> Unit
#
A lambda function triggered when the professional list is loaded.
topBanner: @Composable (() -> Unit)?
#
Sets a top banner for the professional list using a Jetpack Compose composable.
middleDivider: @Composable (() -> Unit)?
#
Sets a custom divider within the professional list using a Jetpack Compose composable.
dividerIndex: Int?
#
Sets the position where the divider should be placed. If null
, the divider is placed over the first inaccessible professional.