Installation
#
Integration ExampleYou have a repository available on Github with a sample project which contains an example of how to integrate our SDK.
#
RequirementsJava | Firebase | MeetingLawyers SDK | android | Status |
---|---|---|---|---|
17+ | 32.7.1+ | 2.1.3 | 23+ (Android 6.0) | Supported |
#
ConfigurationEdit your build.gradle project level:
You need to send us an email to support@meetinglawyers.com to request the android sdk access credentials, and SDK API_KEY
.
Add MeetingLawyers maven repositories to settings.gradle file:
Edit your build.gradle app level:
Include MeetingLawyers SDK lib as a dependency
Make sure the app's build.gradle file contains the following code snippet within android section
#
InitializationEdit or add an application class:
The library must be initialized inside Application.onCreate()
using your API_KEY
, provided by MeetingLawyers.
You can switch between environments by setting the targetEnvironment
parameter to CustomerSdkBuildMode.PROD
or CustomerSdkBuildMode.DEV
.
The build() method returns an instance of MeetingLawyersClient. You can then add this instance to your dependency injection container or setup. This allows the instance to be properly managed throughout the application's lifecycle, improving code maintainability, testing, and scalability.
#
Customization with Builder PatternThe SDK allows UI customization using a builder pattern. You can configure various interface colors before calling build()
.
#
Example Customization#
Available CustomizationsprimaryColor(color: Int?)
Sets the primary interface color.secondaryColor(color: Int?)
Sets the secondary interface color.professionalSpecialityTextColor(color: Int?)
Sets the text color for professional specialties.unreadMessagesBadgeBgColor(color: Int?)
Sets the background color for the unread messages badge.professionalDescriptionColor(color: Int?)
Sets the text color for professional descriptions.disabledProfessionalColor(color: Int?)
Sets the color for disabled professionals.ongoingMessageBgColor(color: Int?)
Sets the background color for outgoing messages.incomingMessageBgColor(color: Int?)
Sets the background color for incoming messages.
#
AuthenticationTo authenticate a patient, provide a valid userId
previously created with the S2S User API.
Make sure not to use any other library method before you receive a successful response in the listener.
#
Push notifications handlingIn order to enable push notifications for chat and video call you must provide MeetingLawyers valid credentials (Server Key and Sender Id) from Firebase Console inside Project settings/Cloud Messaging section:
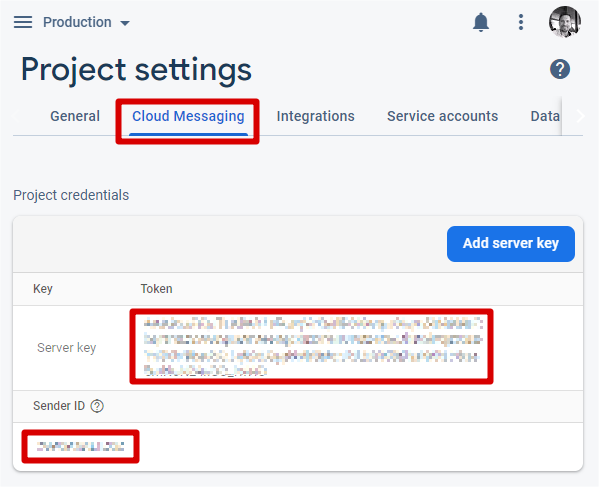
After that, you can proceed to register your push tokens in the SDK:
The SDK will only process its own messages so you can send it all incoming pushes if you can't filter them properly.